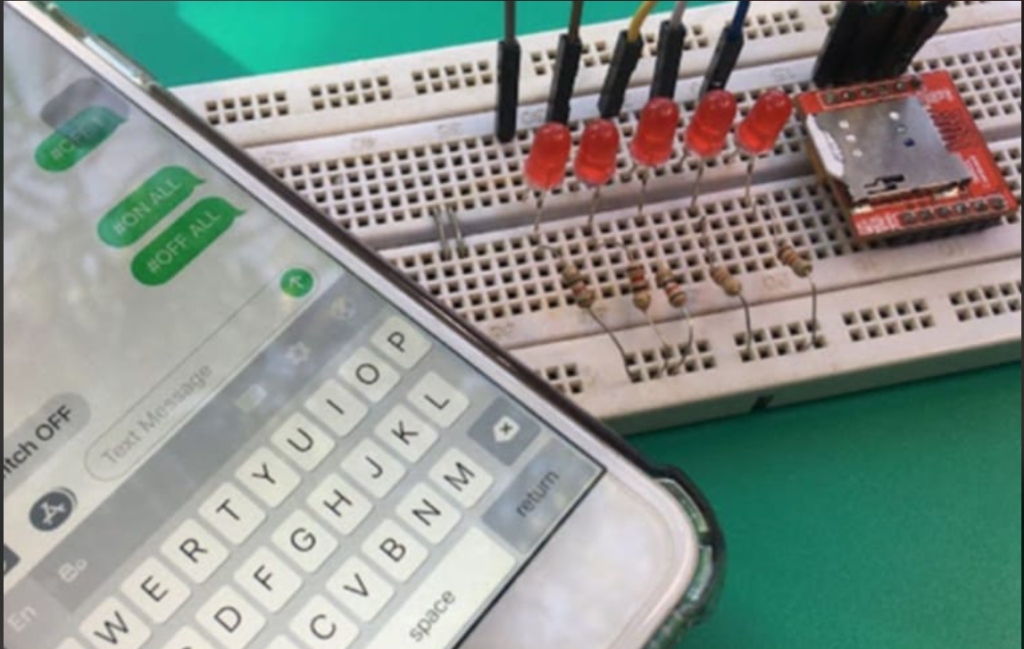
SMS-Controlled Switch System Using Arduino and SIM800L
In this project, we will develop a highly functional SMS-based switching system that uses an Arduino board and a SIM800L GSM module to remotely control the on/off states of various devices. The system allows users to send specific SMS commands from their mobile phones, which are then processed by the Arduino board to execute the desired action. This project is particularly useful in scenarios where remote control of devices is necessary, such as home automation, industrial automation, or agricultural systems.
By implementing this system, users can effortlessly control multiple devices like lights, fans, pumps, or motors from any location with mobile network access. This not only reduces the need for physical interaction but also provides a modern solution for managing devices in remote or inaccessible areas.
In this guide, we will provide a detailed breakdown of the project. We will cover the required components needed to build the system, the installation of libraries necessary for programming the SIM800L module, and an explanation of the Arduino code that powers the functionality. Additionally, we will delve into the working process of the system, illustrating how SMS commands are interpreted and executed. Finally, we will discuss the practical applications of this project, highlighting its utility in various real-world scenarios.
Through this project, you will learn how to integrate GSM technology with microcontrollers, enabling you to create a robust and reliable remote control system. Whether you are a hobbyist or an engineer, this project provides an excellent opportunity to explore the potential of SMS-based communication for automating everyday tasks.
Required Components:
- Arduino Board: 1 unit (e.g., Arduino Uno or Mega)
- SIM800L GSM Module: 1 unit
- Jumper Wires: As needed
- 5V Power Supply: For SIM800L
- Resistors: 1kΩ and 2kΩ (for voltage drop on SIM800L serial lines)
- LED or Devices: 5 units (for testing switches)
- Breadboard or PCB: As required
Library Installation:
We use the Sim800l_EM library in this project. Follow these steps to install it in the Arduino IDE:
- Open the Arduino IDE.
- Go to Sketch > Include Library > Manage Libraries….
- Search for “Sim800l_EM”.
- Select the library and click Install.
Once the library is installed, the code will work seamlessly.
Daigram
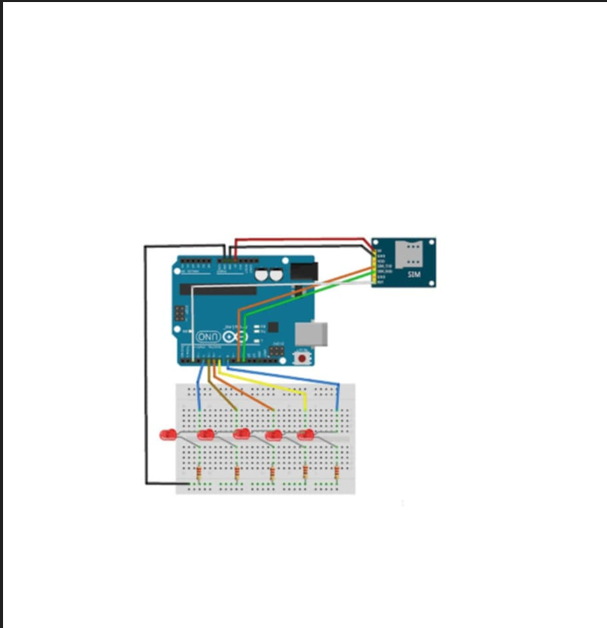
Code
#include <SoftwareSerial.h>
#include <Sim800l_EM.h>
Sim800l Sim800l;
unsigned long bauds = 9600;
String SMScontain; // SMS contain only
String SMSsender; // SMS sender's Name
String text; // Detail SMS
String ReadTXT ="Test" ;
int NUMSMS; //SMS storage
long MSGlen;
uint8_t SMS_QTY;
String dt;
uint8_t index=1;
bool error;
String ReadMsg;
int Switch_1 = 4;// Out Put Pins
int Switch_2 = 5;// Out Put Pins
int Switch_3 = 6;// Out Put Pins
int Switch_4 = 7;// Out Put Pins
int Switch_5 = 8;// Out Put Pins
void setup(){
Serial.begin(bauds);
pinMode(Switch_1,OUTPUT);
pinMode(Switch_2,OUTPUT);
pinMode(Switch_3,OUTPUT);
pinMode(Switch_4,OUTPUT);
pinMode(Switch_5,OUTPUT);
Sim800l.begin();
delay(5000);
Serial.print("Ready to Work");
}
void loop(){
// Get SMS storage amount -------------------------------------
Sim800l.AT();
delay(500);
//Serial.read();
int NUMSMS = Sim800l.getCountsms();
//SMScontain = Sim800l.readMSG(8);
//delay(500);
Serial.println(NUMSMS);
if (NUMSMS !=0 ){
//-------------------------------------------------------------
SMScontain = Sim800l.readMSG(index); // Filter SMS contain
SMScontain.trim();
text = SMScontain;
delay(1000);
ReadTXT = text;
if (text.length() > 0){ // GET SENDER NUMBER
SMSsender = Sim800l.getNumberSms(index);
SMSsender =SMSsender.substring(0,12);
SMSsender.trim();
//Serial.println(SMSsender.length() );
//Serial.println(text);
Sim800l.delAllSms();
// SMSsender = "+12345678910" // If you want to get fead back to a perticular number
//-----------------------------------------------
// FILTER MSG CONTAIN----------------------------
//-----------------------------------------------
if (SMScontain.length() > 0){
Serial.print("SMSsender: ");
Serial.println(SMSsender);
Serial.print("SMS : ");
Serial.println(SMScontain);
}
}
}
//ON OFF by SMS Switch 1----------------------------------
if (SMScontain == "ON 1"){
digitalWrite(Switch_1,HIGH);
error=Sim800l.sendSms(SMSsender,"Switch 01 ON"); // Active if you want a feadback
delay(500);}
else if (SMScontain == "OFF 1"){
digitalWrite(Switch_1,LOW);
error=Sim800l.sendSms(SMSsender,"Switch 01 OFF"); // Active if you want a feadback
delay(500);}
//ON OFF by SMS Switch 2----------------------------------
if (SMScontain == "ON 2"){
digitalWrite(Switch_2,HIGH);
error=Sim800l.sendSms(SMSsender,"Switch 02 ON"); // Active if you want a feadback
delay(500);}
else if (SMScontain == "OFF 2"){
digitalWrite(Switch_2,LOW);
error=Sim800l.sendSms(SMSsender,"Switch 02 OFF"); // Active if you want a feadback
delay(500);}
//ON OFF by SMS Switch 3----------------------------------
if (SMScontain == "ON 3"){
digitalWrite(Switch_3,HIGH);
error=Sim800l.sendSms(SMSsender,"Switch 03 ON"); // Active if you want a feadback
delay(500);}
else if (SMScontain == "OFF 3"){
digitalWrite(Switch_3,LOW);
error=Sim800l.sendSms(SMSsender,"Switch 03 OFF"); // Active if you want a feadback
delay(500);}
//ON OFF by SMS Switch 4----------------------------------
if (SMScontain == "ON 4"){
digitalWrite(Switch_4,HIGH);
error=Sim800l.sendSms(SMSsender,"Switch 04 ON"); // Active if you want a feadback
delay(500);}
else if (SMScontain == "OFF 4"){
digitalWrite(Switch_4,LOW);
error=Sim800l.sendSms(SMSsender,"Switch 04 OFF"); // Active if you want a feadback
delay(500);}
//ON OFF by SMS Switch 5----------------------------------
if (SMScontain == "ON 5"){
digitalWrite(Switch_5,HIGH);//error=Sim800l.sendSms(SMSsender,"Switch 05 ON"); // Active if you want a feadback
delay(500);}
else if (SMScontain == "OFF 5"){
digitalWrite(Switch_5,LOW);
error=Sim800l.sendSms(SMSsender,"Switch 05 OFF"); // Active if you want a feadback
delay(500);}
//ON OFF by SMS all
if (SMScontain == "ON ALL"){
digitalWrite(Switch_1,HIGH);
digitalWrite(Switch_2,HIGH);
digitalWrite(Switch_3,HIGH);
digitalWrite(Switch_4,HIGH);
digitalWrite(Switch_5,HIGH);
error=Sim800l.sendSms(SMSsender,"ALL Switch ON"); // Active if you want a feadback
delay(500);}
else if (SMScontain == "OFF ALL"){
digitalWrite(Switch_1,LOW);
digitalWrite(Switch_2,LOW);
digitalWrite(Switch_3,LOW);
digitalWrite(Switch_4,LOW);
digitalWrite(Switch_5,LOW);
error=Sim800l.sendSms(SMSsender,"ALL Switch OFF"); // Active if you want a feadback
delay(500);}
//Clear Data
SMSsender ="";
SMScontain="";
text="";
if (NUMSMS >1){
Sim800l.delAllSms();}
} //END Void Loop
Code Explanation:
1. Initial Setup:
#include <SoftwareSerial.h>
#include <Sim800l_EM.h>
This section imports the necessary libraries for SIM800L and serial communication.
Sim800l Sim800l;
unsigned long bauds = 9600;
Here, we create a Sim800l
object and set the baud rate for communication.
2. SMS Processing:
int NUMSMS = Sim800l.getCountsms();
This function retrieves the number of SMS messages stored in the module’s inbox.
SMScontain = Sim800l.readMSG(index);
This function reads a specific SMS based on the given index.
3. Device Control:
if (SMScontain == "ON 1") {
digitalWrite(Switch_1, HIGH);
error = Sim800l.sendSms(SMSsender, "Switch 01 ON");
}
If the command "ON 1"
is received, the first switch turns on, and a confirmation SMS is sent.
4. Controlling Multiple Switches Simultaneously:
if (SMScontain == "ON ALL") {
digitalWrite(Switch_1, HIGH);
digitalWrite(Switch_2, HIGH);
digitalWrite(Switch_3, HIGH);
digitalWrite(Switch_4, HIGH);
digitalWrite(Switch_5, HIGH);
error = Sim800l.sendSms(SMSsender, "ALL Switch ON");
}
Commands like "ON ALL"
or "OFF ALL"
will turn all switches on or off simultaneously.
Why Is This Project Important?
1. Remote Control:
You can turn devices on or off by sending an SMS from anywhere. This is highly effective for industrial automation or home automation.
2. Easy Integration:
Using an Arduino and a SIM800L module, you can create a cost-effective system for various applications.
3. Practical Applications:
- Controlling home lights and fans.
- Activating pumps or motors in agriculture.
- Monitoring and controlling security systems.
How This Project Works:
- The user sends a specific SMS command (e.g.,
"ON 1"
) to a designated number. - The SIM800L module receives the SMS and forwards it to the Arduino.
- The Arduino processes the SMS and activates or deactivates the corresponding switch.
- Upon successful execution, the Arduino sends a confirmation SMS back to the sender.
2 thoughts on “SMS-Based Switching System Using Arduino and SIM800L – Complete Guide”